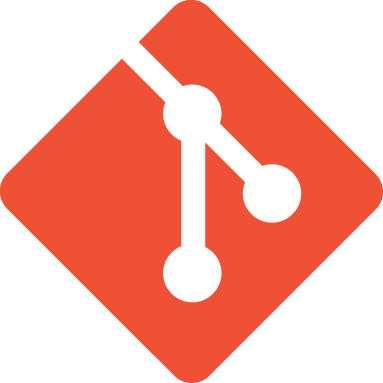
Git
Git is a free and open source distributed version control system designed to handle everything from small to very large projects with speed and efficiency.
Links, Courses and Tutorials about Git.
Windows
macOS
Linux
Visit https://git-scm.com/download/win
After installation above, verify that
git
is installed:Terminal
Output
git --version
The output should now show a version, for example
2.39.0
.Create Repository
Terminal
git init
Add Files
Terminal
git add .
This will add all files in the current working directory to the Git index. Files that were already added won't be added again.
Commit Files
Terminal
git commit -m "Summary of my changes"
After adding your files via
git add
, you still need to commit them. If you want to combine git add
and git commit
, you can use:Terminal
git commit -am "Summary of my changes"
Push files
Pushing your files to Github requires you to create a remote first. After creating a repository on Github, copy its url and run:
Terminal
git remote add origin https://github.com/username/repository.git
You only have to do this once. Then, you can publish your committed changes by running:
Terminal
git push -u origin main
In subsequent pushed, you can shorten the command:
Terminal
git push
Branches
Branches are a core concept of Git. By default, you are on the
main
branch, which is where your production code is hosted. But in software development, features are often worked on in parallel and iteratively. That's why it's recommended to create an isolated branch for your feature:Terminal
git checkout -b my-feature
This will create a new branch
my-feature
and check it out. To switch back to main
, simply run:Terminal
git checkout main
And to switch back to feature, run:
Terminal
git checkout my-feature
Merging
Let's say you made various commits and pushed on the
my-feature
branch. The feature is done, and you want to take it to production. Here's how you can do it:Terminal
git status # on branch my-feature, working tree cleangit checkout main # switch to maingit merge my-feature # merge my-feature into maingit push origin main # push the my-feature merge to maingit branch -d my-feature # delete the my-feature branch
Syncing
So far, we looked at you pushing new changes to Git. But what if you co-worker pushed something, and you want to "download" it?
First, make sure that you are on a clean working tree:
Terminal
git status # on branch my-feature, working tree clean
If it says "working tree clean", you can safely continue. If not, you need to commit your changes first, or stash them:
Terminal
git commit -am "Finish my current step" # commit your changes# orgit stash # save your changes for later
Run
git status
again, until your working tree is clean. Then, pull the latest changes from the remote:Terminal
git pull # pull the changes of my co-workers
If you ran
git stash
before, you can now "drop your changes" on top of the freshly pulled changes:Terminal
git stash apply # add my changes on top of the pulled changesgit commit -am "Finish my current step" # commit your changesgit push # push your changes to the remote, so that others can pull it
Continue my work later
Terminal
git stash # save your changes for latergit pull # pull the changes of my co-workersgit stash apply # add my changes on top of the pulled changes
Undo the last local commit
Terminal
git commit -m "Something terribly misguided" # your mistakegit reset HEAD~ # undo the last commit# ... make correctionsgit add . # add your changes againgit commit -c ORIG_HEAD # commit again, keeping the original message
Credits: Stackoverflow
Github Desktop
If you prefer to use a Graphical User Interface to manage your Git repositories, give Github Desktop a try.