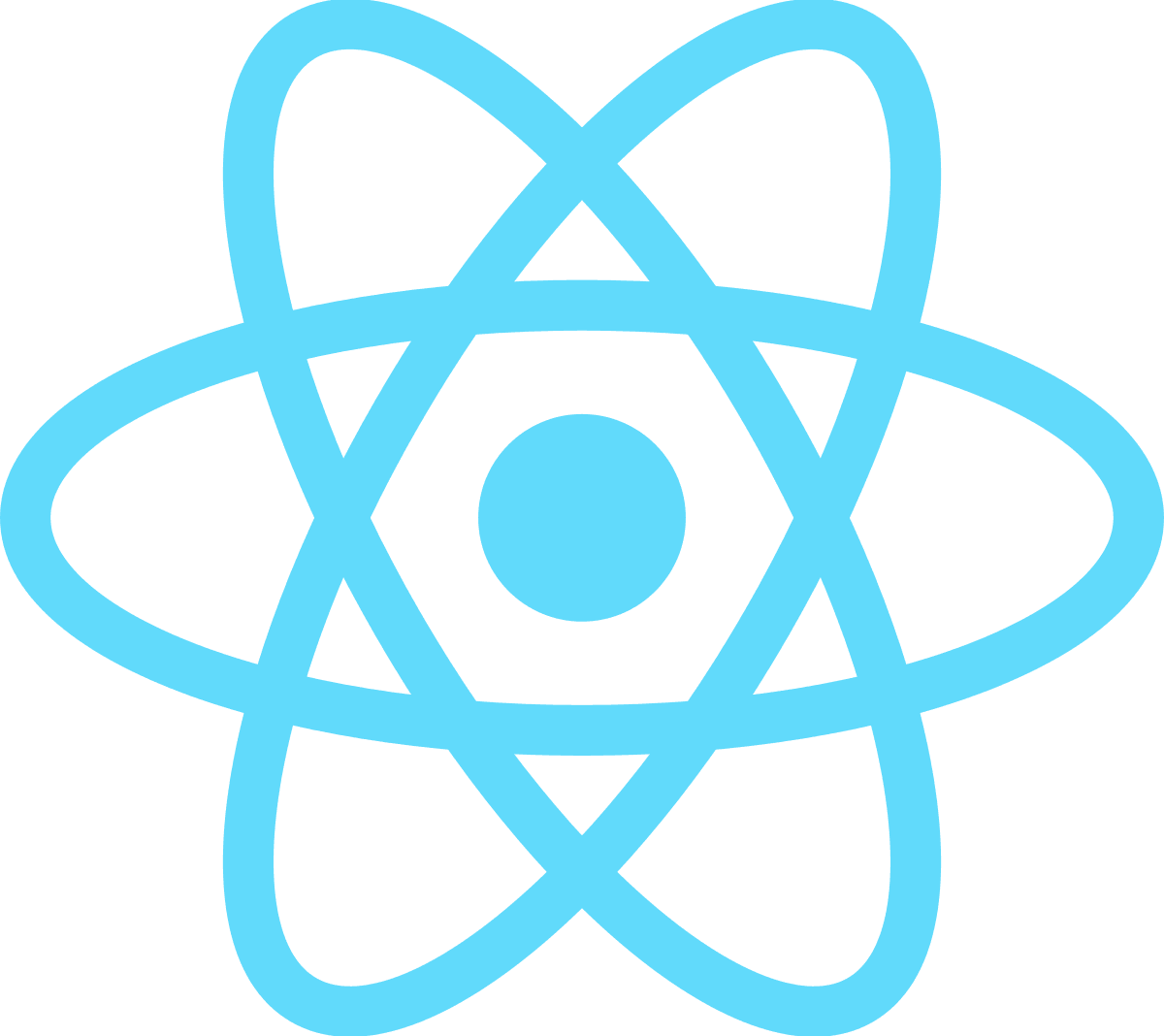
React
The library for web and native user interfaces
Links, Courses and Tutorials about React.
React themselves recommend to use a production grade framework:
Next.js
Remix
Gatsby
Expo
npx create-next-app
Let's quickly dive into the different technologies:
- Next.js is a full-stack React framework that builds on top of React's latest APIs.
- Remix is another full-stack React framework with similar awesome features.
- Gatsby is a React framework for fast CMS-backed websites.
- Expo is a React framework that lets you create universal Android, iOS, and web apps with truly native UIs.
If add React from scratch or to an existing project, you can refer to this guide.
Hooks
useState
State is the core of React's architecture. It's simply a piece of data that can change by user input:
component.tsx
function Counter() { const [count, setCount] = useState<number>(0) return ( <div> <p>You clicked {count} times</p> <button onClick={() => setCount((prev) => prev + 1)}>Increment</button> </div> )}
useEffect
Effects are for side effects that can not be directly handled in the event handler:
component.tsx
function Component() { const [count, setCount] = useState<number>(0) useEffect(() => { console.log(`Count changed! New count: ${count}`) // we want to log to the console (side effect) }, [count]) // every time the count changes return ( <div> <p>You clicked {count} times</p> <button onClick={() => setCount((prev) => prev + 1)}>Increment</button> </div> )}
Effects are often overused though. You might not need an effect.
More hooks
Check this beautiful video from Fireship for a quick intro on all React hooks: