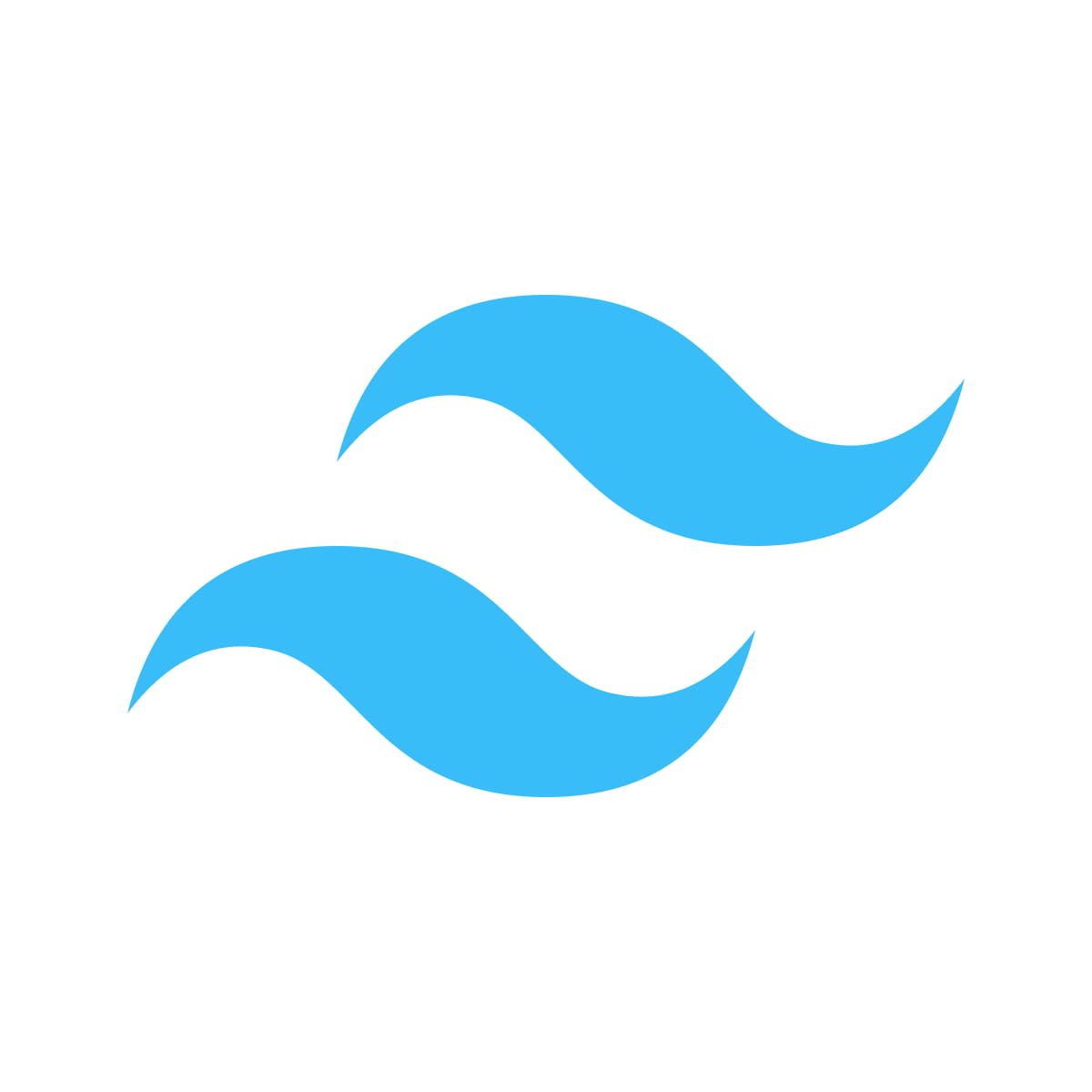
Tailwind CSS
A utility-first CSS framework for rapidly building custom user interfaces
Links, Courses and Tutorials about Tailwind CSS.
Get help with Tailwind CSS from the community or creators.
Many modern frameworks like Next.js already have Tailwind support. In case you would like to set it up manually though, here are the steps:
Terminal
npm install -D tailwindcss postcss autoprefixer
Next, initialize Tailwind:
Terminal
npx tailwindcss init -p
Open
tailwind.config.js
and make sure that the content
field contains all paths that might contain Tailwind classes:tailwind.config.js
/** @type {import('tailwindcss').Config} */module.exports = { content: [ './app/**/*.{js,ts,jsx,tsx,mdx}', './pages/**/*.{js,ts,jsx,tsx,mdx}', './components/**/*.{js,ts,jsx,tsx,mdx}', // Or if using `src` directory: './src/**/*.{js,ts,jsx,tsx,mdx}', ], theme: { extend: {}, }, plugins: [],}
That is because Tailwind generates classes on the fly to keep your bundle size low. This is also the reason why you explicitely need to write out Tailwind classes:
home/page.tsx
const dynamicColor: 'black' | 'white' = 'white'const wrongClass = `bg-${dynamicColor}` // WRONG! Tailwind can not dynamically create classesconst rightClass = { white: 'bg-white', black: 'bg-black' }[dynamicColor] // RIGHT! Explicitely write out classes
Lastly, add the Tailwind declaratives at the top of your main CSS file:
globals.css
@tailwind base;@tailwind components;@tailwind utilities;
Now you can use Tailwind classes in your project:
home/page.tsx
export default function Home() { return <h1 className="text-3xl font-bold underline">Hello world!</h1>}
Plugins
tailwind.config.js
const plugin = require('tailwindcss/plugin')module.exports = { plugins: [ require('@tailwindcss/typography') // adds "prose" class require('@tailwindcss/forms'), // resets form styles require('@tailwindcss/aspect-ratio'), // adds more aspect ratio classes require('@tailwindcss/container-queries'), // adds support for container queries plugin(function({ addVariant }) { // Add your custom plugins here. This example adds support for empty:<class> syntax addVariant('empty', ({ modifySelectors, separator }) => { modifySelectors(({ className }) => { return `.empty${separator}${className}:empty` }) }) }), ]}
Tailwind & VSCode
For more powerful features, like detecting invalid classes or sorting your Tailwind classes, refer to Tailwind's official editor setup guide.